By continuing your visit to this site, you accept the use of cookies by Google Analytics to make visits statistics.
Using Image Search in Your App
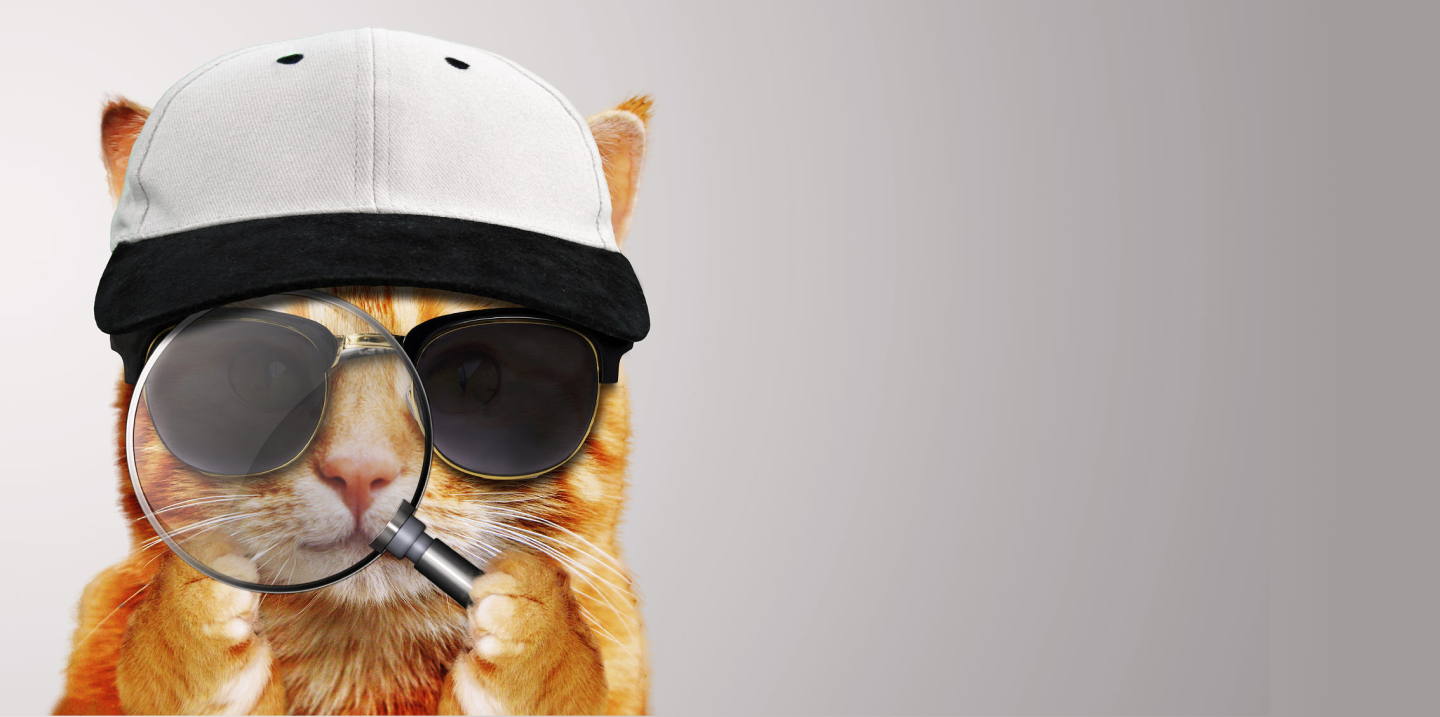
Every content on a site, in an application or simply in a letter, looks more expressive and eye catching when it's accompanied by an image. Do you even remember when you last saw an article without an abstract image? Some are used to great effect, while there is also a practice of inserting a picture “to attract attention” (admit, haven’t you done the same?) which sometimes doesn’t relate to the topic created by the author of the text. Everyone does this, from ordinary users to the media giants, news portals and others. Although the types of images we use may differ, what we can all agree on, is that images are a vital part of our online lives.
Let's say we ask a user to create some content in our system, for example, his financial goal. In this case, it’s necessary to put an image here (to provide an example of the user’s motivation, visualization).
Most of the time, the user will skip this phase, since there are +N steps involved in finding/selecting/taking a photo and uploading it. (That is if a photo is not located somewhere in a desktop or photo gallery, which is often the case - people are lazy).
Fortunately there are lots of solutions that we can help with - For example, you can use a third party service like unsplash or splashbase
Although these are very good services, most likely you will have to pay for them. This can be frustrating when many of the features that come with a paid service may not even be needed (for instance, the number of likes on this photo, the exposure settings, etc.).
If you are already working with some Google products in your project, why not utilize their search service? After all, if you need some pictures, the first place you will go is often a search engine. According to statistics from 2018, 90.15% of users will use Google, so let's do it instead of the user!
First, we need to add the necessary packages if they haven’t been added yet:
pip install google-api-python-client google-auth-httplib2 google-auth-oauthlib
Don't forget to add these packages to the requirements:
pip freeze > requirements.txt
Next, you need to activate the custom search in your account for which you will receive a key(API_KEY). As how to do this is not directly the subject of the article, we point you to Google or this resource below, which will help you if you need assistance:
When creating a Custom Search Engine ID (CSE_ID), the easiest way is to use a panel https://cse.google.com/cse/all (be sure to turn on the image search function)
After the above steps have been taken, we can then create our service class, which will receive a phrase or term for entry (in our case, the goal header). In return, it will show an array of a number of images (you never know if the first one will satisfy the user).
from googleapiclient.discovery import build
from django.conf import settings
API_KEY = getattr(settings, 'GOOGLE_API_KEY')
CX_ID = getattr(settings, 'GOOGLE_CSE_ID')
class GoogleImageSearchService:
"""The service that search images"""
def __init__(self):
self.service = build("customsearch", "v1", developerKey=API_KEY)
def search(self, term):
try:
res = self.service.cse().list(
q=term,
searchType='image',
imgType='photo',
imgSize='xxlarge',
cx=CX_ID,
).execute()
images = res.get('items', [])
response = []
if len(images):
for i in range(10):
image_link = images[i].get('link', '')
if len(image_link):
response.append(image_link)
except Exception as e:
import traceback
print(f"google api error\nterm: {term}\n{str(e)}\n{traceback.format_exc()}")
response = []
return response
Supported Arguments
The Google Custom Search service supports many parameters which you can find in the official documentation.
We used only a few for our task:
searchType='image',
imgType='photo',
imgSize='xxlarge',
The searchType parameter indicates that we are looking for an image - everything is simple here.
Options are available for imgSize - icon, small, medium, large, xlarge, xxlarge, or huge. We tried all these image size options and found xxlarge to be the one that returns the most relevant images by the entered term. We don’t need an icon, this is the goal! :)
Сlipart, face, lineart, stock, photo and animated are available for imgType. The photo option is the most suitable for us.
Of the remaining available parameters, imgDominantColor is interesting; you can specify the predominant color in the photo, but whether you need this feature is up to you.
The pagination parameters were skipped intentionally as 10 images are given by default. This is exactly what we need for the user to choose (even less, but no more)
We return only the links, then depending on the selected image, we will process it as we need (save, optimize, crop, etc.).
And that’s all, the user enters the title, and we can provide an excellent accompanying picture:
The result will differ from what the user would get when using the browser, as the browser has different search settings by default.
If you wish to know more, contact our knowledgeable specialists and we’ll get back to you ASAP!